Throttling Immediate TextBox Binding Behavior for Windows Phone
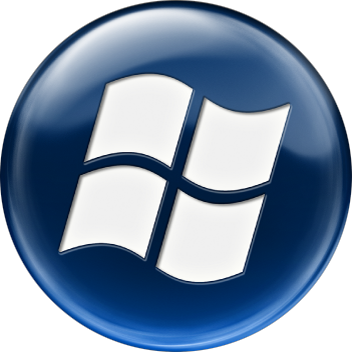
The learning curve of a Windows Phone developer
While developing Offline Web Browser for Windows Phone i had to build a small MVVM framework in order to keep the logic out of the views.
One of the first thing i found missing was a way to force immediate propagation of text entered in TextBox control to the databound property of my ViewModel.
By default, TextBox binding is triggered only when control loses focus, and this is kind of lame.
Code reuse is not a myth!
This is really old problem. It existed in desktop Silverlight from the beginning. And it made its way to the Windows Phone platform.
Fortunately I remembered that back in the days while experimenting with Silverlight MVVM framework i already solved this problem by creating a custom silverlight TextBox control so i decided to just reuse that code and create a Behavior that will force binding update to fire on each keystroke in TextBox.
That’s the beauty of developing a Windows Phone app – you can reuse most of the Silverlight code you built over the years 🙂
But then i noticed another problem. Because now each keystroke was propagating changes to my ViewModel, if user would type fast and i do for example some web service call on each property change – then i can have too many requests to the web service (one for each keystroke) but in fact i want to do a call only when user stopped typing for a while.
Fortunately, solution is very simple – we will use Reactive Extensions (Rx) since its perfect fit and its already built-in into Windows Phone.
We just need to reference Microsoft.Phone.Reactive.dll from GAC (no additional download needed) and we are half-way there.
OK, but show us some code!
Yes lets see how the code for the ThrottledImmediateBindingBehavior looks like:
using System; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Interactivity; using Microsoft.Phone.Reactive; namespace Roboblob.Mvvm.Behaviors { public class ThrottledImmediateBindingBehavior : Behavior { private BindingExpression _expression; public bool Throttle { get; set; } private double _throttleDelayInSeconds = 0.5; private IDisposable _currentObservable; public double ThrottleDelayInSeconds { get { return _throttleDelayInSeconds; } set { _throttleDelayInSeconds = value; } } protected override void OnAttached() { base.OnAttached(); if (Throttle) { this._expression = this.AssociatedObject.GetBindingExpression(TextBox.TextProperty); var keys = Observable.FromEvent(AssociatedObject, "TextChanged").Throttle(TimeSpan.FromSeconds(ThrottleDelayInSeconds)); _currentObservable = keys.ObserveOn(Deployment.Current.Dispatcher).Subscribe(evt => OnTextChanged(evt.Sender, evt.EventArgs)); } else { this._expression = this.AssociatedObject.GetBindingExpression(TextBox.TextProperty); this.AssociatedObject.TextChanged += this.OnTextChanged; } } protected override void OnDetaching() { base.OnDetaching(); if (Throttle) { if (_currentObservable != null) { _currentObservable.Dispose(); this._expression = null; } } else { this.AssociatedObject.TextChanged -= this.OnTextChanged; this._expression = null; } } private void OnTextChanged(object sender, EventArgs args) { if (_expression != null) { this._expression.UpdateSource(); } } } }
Simple Is Beautiful
Our Binding class has bool property Throttle where we can enable/disable throttling and also a ThrottleDelayInSeconds where we specify after how many seconds later after user stops typing our property is updated.
In the OnAttached – we just hook to the TextChanged and each time user types something we update the binding source.
But if Throttle is set to true, we hook to the TextChanged event over Reactive Extensions and we setup throttling so that binding do not trigger if changes are too fast.
In this only after changes stop firing for the time defined in ThrottleDelayInSeconds, only then binding update is triggered.
Off course we do a little cleanup in the OnDetaching as all good coders 🙂
What about the Xaml???
Yes in Xaml we can use it like this:
<TextBox Text="{Binding SearchCriteriaText, Mode=TwoWay}"> <i:Interaction.Behaviors> <RoboblobBehaviors:ThrottledImmediateBindingBehavior Throttle="True" ThrottleDelayInSeconds="1" /> </i:Interaction.Behaviors> </TextBox>
For those who are lazy to type I’m attaching a zipped source code of simple Windows Phone Mango project that is demonstrating the usage of the behavior.
Btw does somebody even remember how it was to type source code from printed computer magazines int your 8bit computer?
Probably not 😀
Well at least I hope that someone will find this useful.
Until next time, happy coding!
Hello.
I’m not sure I quite understand the point of this workaround. Why not simply set the UpdateSourceTrigger of the particular binding to ‘PropertyChanged‘? This way, the ‘SearchCriteriaText’ gets updated every time the ‘Text’ property on the TextBox changes.
Hi Emir,
probably you should download the zipped solution at the end of the post and run it to see the difference.
Changes in TextBox are being throttled so that we don’t trigger the underlying databound property on each key stroke, but only after user stopped typing.
You usually want to throttle events if the handler code is CPU expensive and/or lenghty so it does not make sense to invoke it each time value changes, but only when we are sure that the value stopped changing.
Hope this clarifies it.
Thanks for your feedback!
Thank you very much!!!
I was looking for de-bounce like in JS for WPF and it helped so much.
The only thing is that I had to adapt some code(line 32,33) to Rx.NET
var keys = Observable
.FromEventPattern(
handler => this.AssociatedObject.TextChanged += handler,
handler => this.AssociatedObject.TextChanged -= handler)
.Throttle(TimeSpan.FromSeconds(ThrottleDelayInSeconds));
_currentObservable = keys.ObserveOn(Dispatcher).Subscribe(evt => OnTextChanged(evt.Sender, evt.EventArgs));