Dot Net Gotcha #1 – List versus Collection constructor
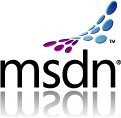
Don’t Google it!
Do you know (without Googling it) what will this console application display when executed?
class Program { static void Main(string[] args) { var originalNumbers = new List<int> { 1, 2, 3, 4, 5, 6 }; var list = new List<int>(originalNumbers); var collection = new Collection<int>(originalNumbers); originalNumbers.RemoveAt(0); DisplayItems(list, "List items: "); DisplayItems(collection, "Collection items: "); Console.ReadLine(); } private static void DisplayItems(IEnumerable<int> items, string title) { Console.WriteLine(title); foreach (var item in items) Console.Write(item); Console.WriteLine(); } }
If you try to think logically, you expect that both List and Collection will contain numbers from 1 to 6 when they are displayed, right?
Wrong, otherwise this would not be a proper gotcha 😉
Here is the actual output of the application (surprise, surprise):
Do you know why?
Answer is actually very simple. If you check the MSDN documentation for Collection constructor that accepts generic IList you will see this text:
Initializes a new instance of the Collection class as a wrapper for the specified list.
That means that this constructor creates just a wrapper around the existing IList, so adding or removing items to the original list will also affect the new Collection we just created, because its just a wrapper and uses original List under the hood.
On the other hand List constructor that accepts IEnumerable<T> behaves properly and does what you would logically expect it to do:
Initializes a new instance of the List<T> class that contains elements copied from the specified collection and has sufficient capacity to accommodate the number of elements copied.
So when you create a new List and pass an existing List to its constructor it will create a proper new empty list, and then copy all the references from original List to the new List.
Because of that, the new List is ‘detached’ from the original and this is why it remains unchanged even if we modify the original List.
Moral of the story
This is simply how Microsoft made these two classes (i would not say its very consistent or good but who am i to judge, right?) and as long as you know it – its fine.
And if you don’t know this – it can bite you really hard.
All that you can do is to try to remember this, and also: RTFM.
Btw, did i said that this can be an excellent job interview question? 😉
Until next Dot Net Gotcha, keep reading those manuals…
Great tip. Thanks!